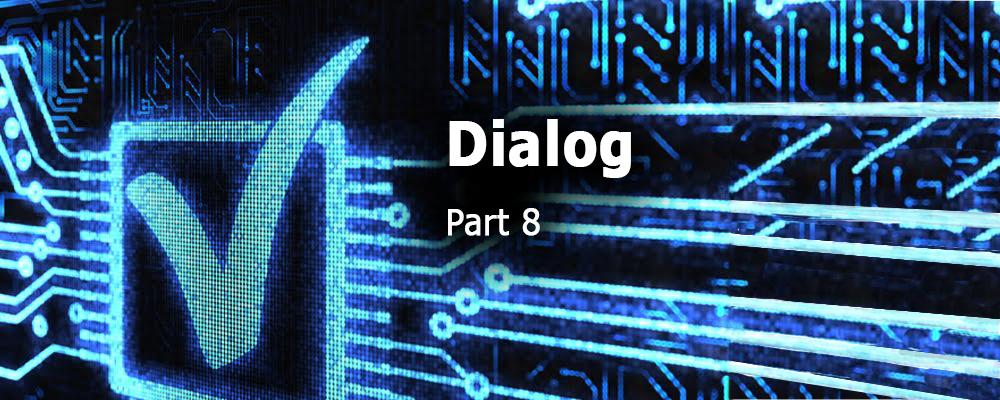
Creating QML Controls From Scratch: Dialog
Continuing our QML Controls from Scratch series, this time we will implement a Dialog that supports an arbitrary number of Buttons (and we reuse our Button control).
Its public API includes the text to show, an array of strings for the buttons, and a clicked() signal that provides the index of the button the user clicked. Unlike other controls in the series, Dialog is full screen so we place it at the root level in Test.qml and at the bottom so it is highest in z order (i.e. on top).
The client code (in Test.qml) takes responsibility for managing the visible property of the Dialog. We need to provide a MouseArea to prevent touches on anything but the Buttons from passing through to (hidden) controls beneath the Dialog.
Dialog.qml
import QtQuick 2.0
Rectangle { // white background
id: root
// public
property string text: 'text'
property variant buttons: []//'0', '1']
signal clicked(int index); //onClicked: print('onClicked', index)
// private
width: 500; height: 500 // default size
MouseArea{anchors.fill: parent} // don't allow touches to pass to MouseAreas underneath
Text { // text
text: root.text
anchors{centerIn: parent; verticalCenterOffset: -0.1 * root.height}
font.pixelSize: 0.1 * root.height
width: 0.9 * parent.width
wrapMode: Text.WordWrap
horizontalAlignment: Text.AlignHCenter
}
Row { // buttons
id: row
anchors{bottom: parent.bottom; horizontalCenter: parent.horizontalCenter; bottomMargin: 0.1 * root.height}
spacing: 0.03 * root.width
Repeater {
id: repeater
model: buttons
delegate: Button {
width: Math.min(0.5 * root.width, (0.9 * root.width - (repeater.count - 1) * row.spacing) / repeater.count)
height: 0.2 * root.height
text: modelData
onClicked: root.clicked(index)
}
}
}
}
Test.qml
import QtQuick 2.0
Dialog {
id: dialog
text: 'Are you sure?'
buttons: ['No', 'Yes']
onClicked: visible = false
}
Summary
Hopefully, this post has helped you master Dialog. In the next installment in the Creating QML Controls from Scratch series, we'll create PageDots. The source code can be downloaded here. Be sure to check out my webinar on-demand. I walk you through all 17 QML controls with code examples.