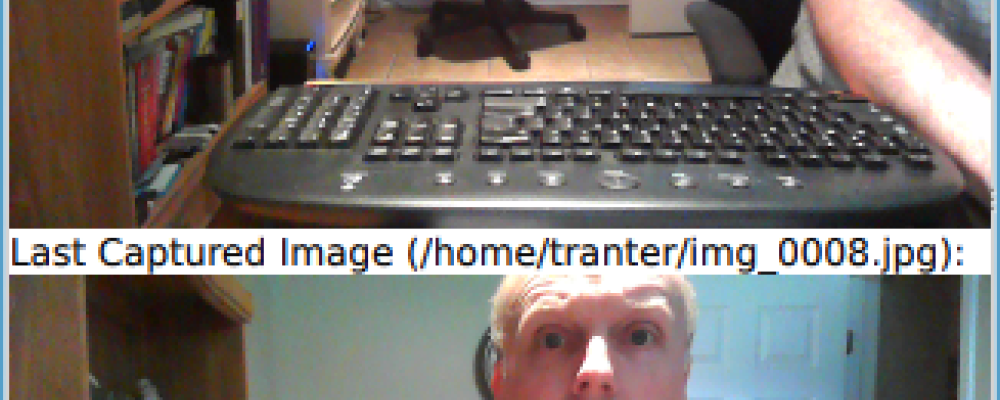
The Qt 5 Multimedia QML Elements
Introduction
Qt 5 introduced a new set of multimedia APIs. Both C++ and QML APIs are provided. In this blog post, I will give an overview of the QML APIs for multimedia.
Multimedia QML Elements
The QtMultimedia module provides the following QML components:
Name | Description |
---|---|
Video | A convenience type for showing a specified video |
Audio | Add audio playback to a scene |
MediaPlayer | Add media playback to a scene |
Camera | Access viewfinder frames and take photos and movies |
CameraCapture | An interface for capturing camera images |
CameraExposure | An interface for exposure related camera settings |
CameraFlash | An interface for flash related camera settings |
CameraFocus | An interface for focus related camera settings |
CameraImageProcessing | An interface for camera capture related settings |
CameraRecorder | Controls video recording with the Camera |
Radio | Access radio functionality |
RadioData | Access RDS data |
Torch | Simple control over torch functionality |
VideoOutput | Render video or camera viewfinder |
SoundEffect | Provides a way to play sound effects |
A related module, QtAudioEngine, provides support for 3D positional audio playback and content management. It defines another nine QML elements. We won't cover that module in this blog post.
How to Use
To use the module, you need to include the QtMultimedia module version 5.0. Typically, you will also need to import QtQuick version 2 or newer, e.g.
import QtQuick 2.0 import QtMultimedia 5.0
Qt Multimedia uses each platform's underlying native multimedia system on the back end. It uses DirectShow and WMF on Windows, AVFoundation on Mac and GStreamer on Linux. The audio and video codecs that are supported are determined by what is installed and supported by the back end on your system.
Compatibility with Qt 4
Qt Multimedia in Qt 5 replaces the Qt Multimedia module from Qt 4 and the Qt Multimedia Kit module from Qt Mobility. It is reasonably compatible with Qt Multimedia from Qt 4 at the source level. It is less compatible with Qt Multimedia Kit. The section Changes in Qt Multimedia in the Qt documentation describes the changes in more detail.
Audio
Here is a simple first example that plays a sound file when text is clicked. It uses the Audio element:
import QtQuick 2.0 import QtMultimedia 5.0 Text { text: "Press Me!" font.pointSize: 24 Audio { id: playMusic source: "/usr/share/sounds/pop.wav" // Point this to a suitable sound file } MouseArea { anchors.fill: parent onPressed: playMusic.play() } }
The Audio element can play sound files. It provides a number of properties including access to sound metadata, signals that are emitted when events of interest occur (e.g. playbackStateChanged()) and methods to control playback.
A similar element, SoundEffect, can play uncompressed sound files like WAV files and generally has lower latency than the Audio element but can play a smaller range of sound files. Here is the example above done using SoundEffect, the only difference being the Audio element is replaced with SoundEffect:
import QtQuick 2.0 import QtMultimedia 5.0 Text { text: "Press Me!" font.pointSize: 24 SoundEffect { id: playMusic source: "/usr/share/sounds/pop.wav" // Point this to a suitable sound file } MouseArea { anchors.fill: parent onPressed: playMusic.play() } }
Video
For video playback, you can use the MediaPlayer element to define and control playback of a video or sound file. It is a non-visual component, so you then need to use a VideoOutput element to render the content to the screen. Here is an example:
import QtQuick 2.0 import QtMultimedia 5.0 Rectangle { width: 480 height: 270 MediaPlayer { id: player source: "trailer_1080p.mov" // Point this to a suitable video file autoPlay: true } VideoOutput { source: player anchors.fill: parent } }
There is also a convenience element Video that combines the functionality of MediaPlayer and VideoOutput that will often suffice when doing simple video playback functionality. Here is an example similar to the previous one but using Video:
import QtQuick 2.0 import QtMultimedia 5.0 Video { id: video width: 480 height: 270 autoPlay: true source: "trailer_1080p.mov" // Point this to a suitable video file }
All of these elements have properties, signals and methods, which allow you to build a more complete media player, for example. You can even use a VideoOutput as the source for graphical effects from the QtGraphicalEffects module.
Camera
Most mobile devices, tablets and even laptops now include cameras. The Camera element allows you to capture images and video from a camera with various capture and processing settings. You can use a VideoOutput with the camera as a source to display live video from the viewfinder in your application.
Here is a simple example that shows live preview from a camera:
import QtQuick 2.0 import QtMultimedia 5.0 Item { width: 640 height: 480 Camera { id: camera } VideoOutput { source: camera anchors.fill: parent } }
Here is a more complete example, which shows a preview, captures an image when the preview is clicked, and shows the most recent image captured.
import QtQuick 2.0 import QtMultimedia 5.0 Item { width: 320 height: 510 Camera { id: camera imageCapture { onImageCaptured: { // Show the preview in an Image photoPreview.source = preview } onImageSaved: { text.text = qsTr("Last Captured Image (%1):").arg(camera.imageCapture.capturedImagePath) } } } Column { Text { height: 15 text: qsTr("Preview (Click to capture):") } VideoOutput { source: camera focus: visible // To receive focus and capture key events when visible width: 320; height: 240 MouseArea { anchors.fill: parent onClicked: camera.imageCapture.capture() } } Text { id: text height: 15 text: qsTr("Last Captured Image (none)") } Image { id: photoPreview width: 320; height: 240 } } }
Below is a screen shot of the example running.
There are QML types such as CameraFocus and CameraFlash that are available as properties of a Camera element. A separate element, Torch, controls a camera light, which may be present independent of a camera flash.
Radio
The final type of multimedia device supported is a radio tuner, via the Radio QML element. Unlike the hardware described earlier, radio tuners aren't commonly available on desktop system but are present on some tablets and phones.
As well as controlling the radio, on supported hardware the radioData property returns a RadioData element, which provides access to Radio Data System (RDS) information like station and program names for radio stations that broadcast it.
As I don't have any hardware that supports radio, I won't present an example program. The documentation for the Radio element has a simple example you can try if you wish.
Summary
With Qt 5, it is easy to integrate multimedia from within QML applications. The Qt documentation covers all of the QML elements as well as the C++ multimedia APIs. The Qt source also includes a number of QML examples using multimedia. A future blog post may cover the C++ multimedia APIs.