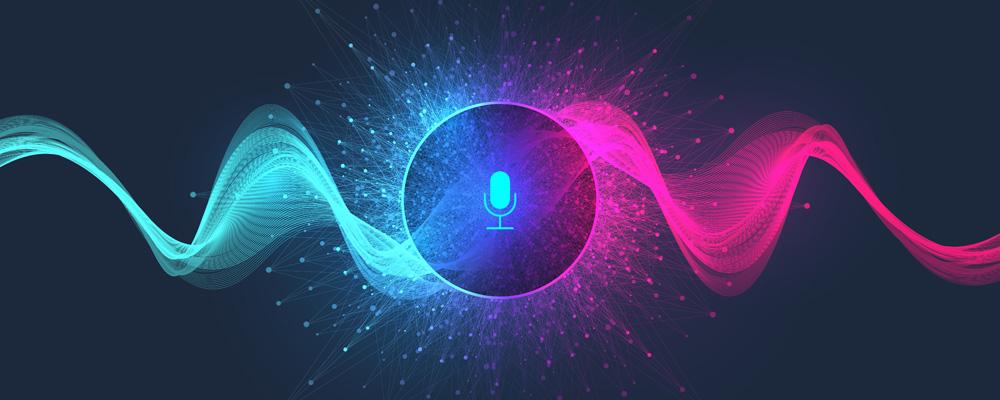
It's Baaack! Qt Speech Returns for Qt 6.4.0 Release
The Qt Speech module was introduced in Qt 5.8.0. I made a blog post at that time (June 2017), looking at how it provided cross-platform support for text-to-speech. With the Qt 6.0.0 release, Qt Speech was one of the modules that were no longer available. In the upcoming Qt 6.4.0 release, it is again going to be part of Qt.
Features
QtSpeech provides cross-platform support for real-time text-to-speech output. It uses platform-specific back ends to do the actual speech generation and is supported on the Linux, macOS, Android, and Windows platforms.
Common use cases for text-to-speech are to support visually impaired users, applications where users cannot use a touchscreen or keyboard like in-vehicle applications, or any application where speech output might augment the user interface.
It provides both C++ and QML interfaces.
Depending on the platform-specific back end, it has support for different locales (language, country, text encoding), different voices (typically having name, gender, and age) and you can set the pitch, rate, and volume of playback.
It has a standard Qt interface with properties, signals, and slots and runs in the background in a non-blocking fashion.
C++ API
The C++ interface consists of the classes QTextToSpeech and QVoice, which are covered in the Qt documentation. We'll look at a simple example shortly.
QML API
A QML type called TextToSpeech is also provided to support using the module from QML code. As of Qt 6.4.0 Beta 2, it is not yet fully documented in the Qt documentation, but it is straightforward to use and we'll also look at an example.
Differences from Qt 5
The Qt Speech module in Qt 6 is almost fully source compatible with the Qt 5 version, so it should be simple to port existing code.
There are some new methods and properties. The QTextToSpeechEngine and QTextToSpeechPlugin classes that were in Qt 5 are still present, but are no longer considered part of the public API and are subject to change.
The TextToSpeech QML type is new in Qt 6 and was not present in Qt 5.
Building
To use the Qt Speech module in your application, you will need to add this line to your project if using qmake:
QT += texttospeech
And these lines to your CMakeLists.txt file if using CMake:
find_package(Qt6 REQUIRED COMPONENTS TextToSpeech)
target_link_libraries(mytarget PRIVATE Qt6::TextToSpeech)
A Simple Code Example (C++)
Here is a simple example of a small but complete program that uses QTextToSpeech. We create a QTextToSpeech object, set the locale, and use the say() method to output some speech. Other properties can be set, but we rely here on the defaults.
#include <QTextToSpeech>
#include <QThread>
int main()
{
// Create an instance using the default engine/plugin.
QTextToSpeech *speech = new QTextToSpeech();
// Set locale.
speech->setLocale(QLocale(QLocale::English, QLocale::LatinScript, QLocale::UnitedStates));
// Say something.
speech->say("Hello, world! This is the Qt speech engine.");
// Wait for sound to play before exiting.
QThread::sleep(10);
}
Simple Code Example (QML)
And here is a small QML example that can be run using the qml program. It creates a TextToSpeech element and calls its say() method when the user clicks on a MouseArea contained in a Rectangle.
import QtQuick
import QtTextToSpeech
Rectangle {
width: 200
height: 200
Text {
anchors.centerIn: parent
text: "Click to Speak"
}
TextToSpeech {
id: tts
volume: 1.0
pitch: 0.5
rate: 0.3
}
MouseArea {
anchors.fill: parent
onClicked: {
tts.say("Hello, world!")
}
}
}
Qt Speech Examples
The Qt source code ships with two Qt Speech examples - one with C++/widgets and one QML. These can be found within the Qt Creator IDE and illustrate most of the features of the module.
Other Notes and Tips
Depending on the platform, Qt Speech has support for different languages so you need to set the locale and character encoding in order for it to correctly generate speech for a given language. The languages and available voices vary with the platform.
The speech quality also varies on each platform. Linux uses open-source text-to-speech libraries which tend to be lower in quality than on the platforms that have commercial speech generation engines built into the operating system.
Summary
The Qt Speech module makes it easy to add speech output to Qt applications. With the Qt 6.4.0 release it is now back and is available from QML as well as C++. Why not consider using it in your next application?