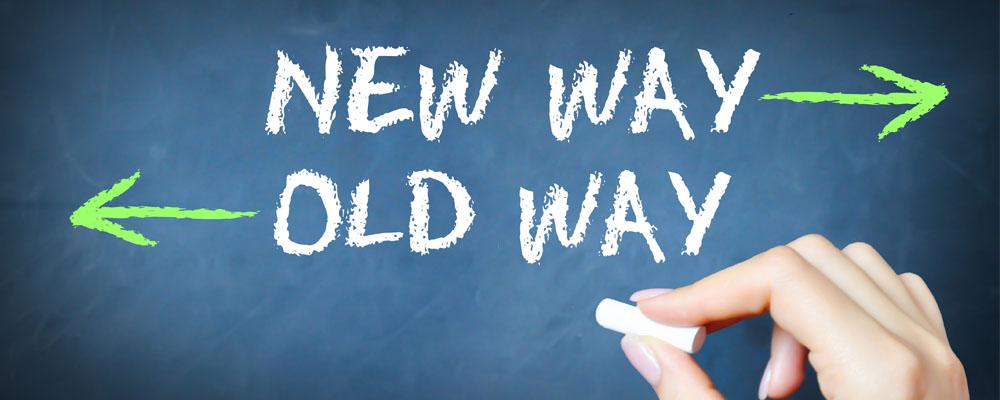
Rust Might be the Right Replacement for C++
For years C and C++ have been the dominant system programming languages, especially for high-performance and embedded applications. While I don't think that will change any time soon, there is an interesting alternative that has been increasingly growing in popularity: Rust. Let's take a look at this up-and-coming programming language and what you need to know about it.
About Rust
Rust is a compiled language, with performance comparable to C++ and a syntax that is also similar to C++. It is suitable for much of the same problem domains as C++: systems programming and the development of large complex systems.
Its main distinguishing characteristic is that it is designed for memory safety without sacrificing performance. It can guarantee memory safety by using a borrow checker to validate references. Unlike some other languages, it does not use garbage collection and reference counting is optional.
Originally developed by Mozilla research, Rust is now run by an independent non-profit foundation. The language does not permit null pointers, dangling pointers, or data races in safe code. Data values can be initialized only through a fixed set of forms, all of which require their inputs to be already initialized.
Rust supports the features of object-oriented programming including types and polymorphism. While strongly typed, it supports type inference for variables declared with the keyword let (similar to C++ auto). Below is some sample code as an example that demonstrates implementing both the ubiquitous hello world function, as well as a function which calculates factorials:
// Hello world plus factorial and for loop
fn factorial(i: u64) -> u64 {
match i {
0 => 1,
n => n * factorial(n-1)
}
}
fn main() {
println!("Hello, World!");
for i in 0..20 {
println!("factorial({}) = {}", i, factorial(i));
}
}
Note the fn keyword which defines a function, accepting a variable of type u64, a standard type for unsigned 64-bit numbers. The arrow defines the return type.
The match keyword is like a switch statement in C or C++, but with some differences such as support for ranges and no fall-through.
Output, done here using println, is an example of calling what Rust calls a macro (indicated by "!").
Rust includes an optional build system called cargo which simplifies building and running of applications and managing dependencies.
Rust supports calling code not implemented in Rust, which it refers to as unsafe code, as the external code can't make the same memory safety guarantees. An example would be calling Qt libraries written in C++. There are currently several Rust bindings and code generators for Qt, with support for both widgets and QML.
A 2021 survey of programmers by SlashData reported that Rust, along with Lua, was one of the two fastest growing programming language communities in the past 12 months.
Linus Torvalds is open to the idea of portions of the Linux kernel being written in Rust and Microsoft has announced a preview of Rust for Windows, which allows creating Rust apps for Windows with bindings for WIN32 APIs. The Google Android team is also interested in using Rust for new development of the OS. Facebook is using Rust in all aspects of development and reports having hundreds of developers writing millions of lines of Rust code.
There is now some support for embedded platforms (including microcontrollers) and the ability to compile to WebAssembly to run Rust code in a web browser.
Two Linux security researchers estimate that about two-thirds of Linux kernel vulnerabilities come from memory safety issues. One Microsoft security expert estimates that 70% of the CVEs originating at Microsoft are memory safety issues. Rust, in theory, can avoid these with its inherently safer memory model.
Rust is a possible replacement for C++ for many applications, with use mostly being driven by Rust's support for memory safety. Comparable to C++ in performance and platform support and controlled by an independent foundation, Rust is definitely a technology worth keeping an eye on. If you want to get your feet wet with Rust, a good starting point is the project web site.